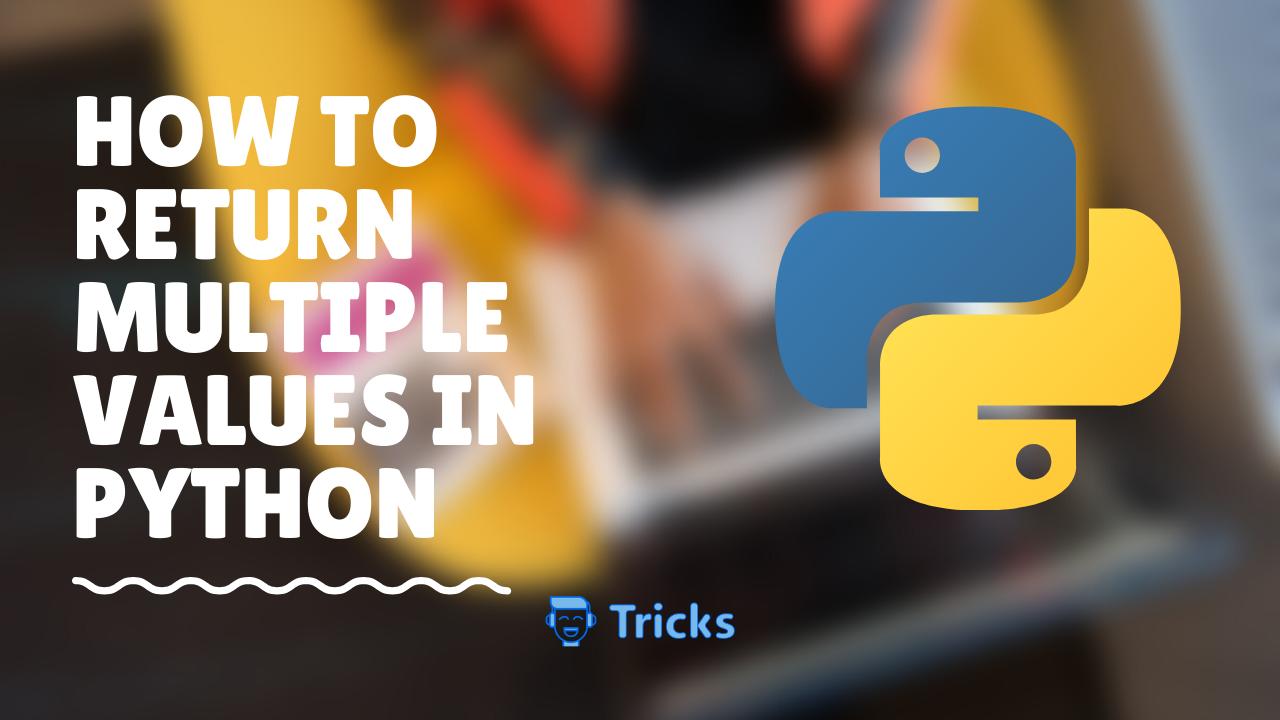
Functions in Python can return multiple values in a form of Python tuple, list, dictionaries or an object of a user-defined class. In this blog, we will show you how you can return values in Python functions easily.
Following are different ways to return multiple values in Python.
1. Using Tuple
A Tuple is an ordered and immutable sequence that can not be changed. Tuple can be created with or without brackets(). Tuples can return multiple values using comma as shown below:
def person():
return "Steve", 25, "London"
print(person())
("Steve", 25, "London")
2. Using a List
A list is an array of items created using square brackets. They are ordered, mutable sequence i.e it can be changed. Here’s an example of how you can return multiple values in python using a list
def five_numbers():
numbers = [] for i in range(1, 6):
numbers.append(i)
return numbers
print(five_numbers())
[1, 2, 3, 4, 5]
3. Using a Dictionery
Dictionaries are ordered collections of data values that can be changeable but do not allow duplicates. It stores data values in key: value pairs. The following example explains how a dictionary can be useful to return multiple values.
def capital_country(capital, country):
location = {}
location[capital] = country
return location
fav_location = capital_country("Tokyo", "Japan")
print(fav_location)
{'Tokyo': 'Japan'}
Using the above ways you can return multiple values in the Python function. You can use any data type based on the program requirement.
If you are working with a small set of values you can use Tuple (comma separated method) or list methods. If you return a larger set of values from a function dictionary method, you can send multiple values back to the primary program.
Let us know if you have any doubt related to the above data types in the comment section below. Thank you for Reading!