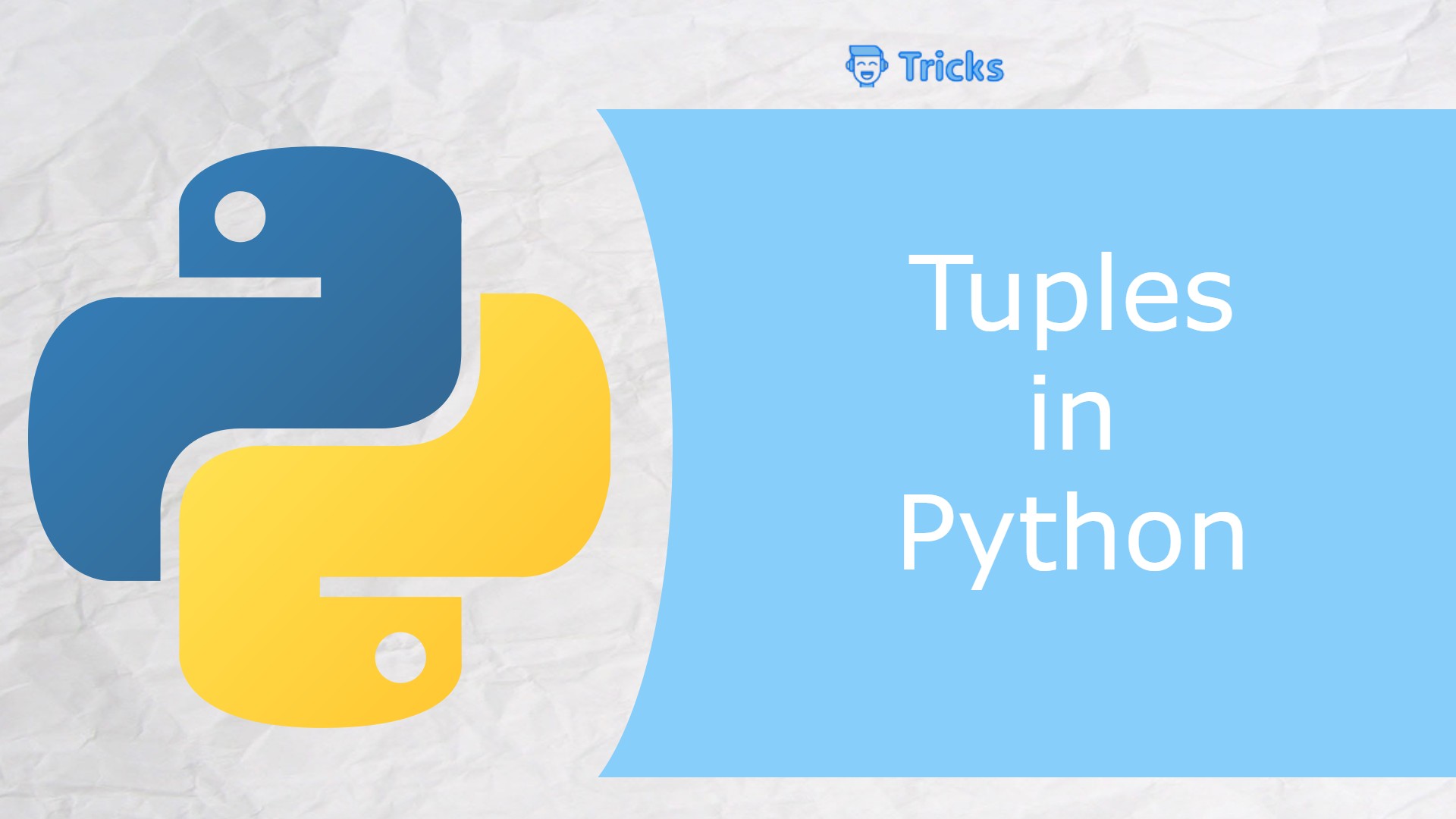
Tuples in Python is a type that is an ordered and immutable collection of objects. Tuples are one of the four built in data types in python that is used to store collections of data.
Tuples are the same as lists in Python but have some properties that make them different. Following are the properties that make them different:
- Unlike Lists that encloses elements in ([]), Tuples are defined by enclosing the elements in parentheses (
()
). - Tuples can not be changed, unlike lists. They are immutable.
Tuples are used to store multiple values in a single variable. Creating a tuple is simple like putting different comma-separated values.
Also read: Basics of git commands explained
Following are different types of Tuples in Python
# Empty tuple
tuple1 = ()
print(tuple1)
# Tuple having integers
tuple2 = (15, 20, 25)
print(tuple2)
# tuple with mixed datatypes
tuple3 = (5, "Hello World", 1.8)
print(tuple3)
# nested tuple
tuple4 = ("Snake", [9, 3, 1], (1, 2, 3))
print(tuple4)
()
(15, 20, 25)
(5, 'Hello', 1.8)
('Snake', [9, 3, 1], (1, 2, 3))
Tuple items are ordered, which means that the items have a defined order, and that order will not change. Unchangeable meaning that we cannot change, add or remove items after the tuple has been created and allow duplicate values.
Tuple items are indexed, the first item has index [0]
, the second item has index [1]
etc.
A tuple can also be created without the parenthesis. For example:
tuple_data = 6, 8.3, "Charlie"
A tuple can be created with a single element. Here you have to add a comma after the element. If we do not put a comma after the element, python will treat the variable as an int variable rather than a tuple.
tuple_data = (8,)
Tuples can be interconnected. This is called Concatenation
tuple_data1 = (0, 1, 2, 3)
tuple_data2 = ('tech', 'python')
print(tuple_data1 +tuple_data2)
(0, 1, 2, 3, 'tech', 'python')
Repetition in Tuples :
tuple_data =('color',)*3
print(tuple_data)
(color, color, color)
Accessing tuple items
To access tuple items we use indexes. Tuple indexes are zero-based, so the first element of a non-empty tuple is always tuple[0]
. We can use the index operator []
to access an item in a tuple.
Note:
- TypeError: If you do not use integer indexes in the tuple. For example, tuple_data[1.0] will raise this error. The index must always be an integer, so we cannot use float or other types
- IndexError: index out of range. This error occurs when we mention the index which is not in the range. For example, if a tuple has 5 elements and we try to access the 7th element, this error would occur.
Accessing tuple elements using positive indexes
As we said, indexes in tuple starts with [0]
and then next element will be [1]
and so on.
tuple_data = ("Python", "Java", "Dart", "Javascript")
# displaying all elements
print(tuple_data)
# accessing the first element
# prints "Python"
print(tuple_data[0])
# accessing third element
# prints "Java"
print(tuple_data[2])
Output:
("Python", "Java", "Dart", "Javascript")
Python
Java
Accessing tuple elements using negative indexes
Python allows negative indexing for its sequences similar to lists and strings.
The index of -1 to access last element, -2 to access second last and so on.
# Negative indexing for accessing tuple elements
my_tuple = ('W', 'a', 't', 'e', 'r')
# Output: 'r'
print(my_tuple[-1])
# Output: 'w'
print(my_tuple[-5])
r
w
Accessing elements from nested tuples
The first index represents the element of main tuple and the second index represent the element of the nested tuple.
In the following example, tuple_data[2][1] accessed the second element of the nested tuple. Because 2 represented the third element of the main tuple which is a tuple and 1 represented the second element of that tuple.
tuple_data = (5, "Python", (Laptop, Tablet, Mobile))
# prints Tablet
print(tuple_data[2][1])
Tablet
Operations that can be performed on tuples in Python
Now that we ae aware of how to access the tuple lets look at how we can use operations on tuples in python.
Changing a Tuple
Tuples are immutable. This means that elements of a tuple cannot be changed once they have been assigned. But, if the element is itself a mutable data type like a list, its nested items can be changed.
tuple_data = (4, 2, 3, [6, 5])
# changing the element of tuple
# This is not valid since tuple elements are immutable
# TypeError: 'tuple' object does not support item assignment
# tuple_data[1] = 9
# However, item of mutable element can be changed
tuple_data[3][0] = 9
# Output: (4, 2, 3, [9, 5])
print(tuple_data)
# Tuples can be reassigned
tuple_data = ('c', 'u', 'r', 'r', 'e', 'n', 'c', 'y')
# Output: ('c', 'u', 'r', 'r', 'e', 'n', 'c', 'y')
print(tuple_data)
(4, 2, 3, [9, 5])
('c', 'u', 'r', 'r', 'e', 'n', 'c', 'y')
Deleting operation in a tuple
As we already know that tuples are immutable, which means elements in a tuple cannot be changed or deleted. However, Deleting the entire tuple is possible by using the operator del
# Deleting elements in tuple
tuple_data = ('p', 'r', 'o', 'g', 'r', 'a', 'm', 'e', 'r')
# can't delete items
# TypeError: 'tuple' object doesn't support item deletion
# del tuple_data[3]
# Can delete an entire tuple del tuple_data
# NameError: name 'tuple_data' is not defined
# This is because the tuple has been deleted above
print(tuple_data)
Traceback (most recent call last): File "<string>", line 12, in <module> NameError: name 'tuple_data' is not defined
Slicing in tuple
We can access a range of items in a tuple by using the slicing operator colon :
tuple_data = (11, 22, 33, 44, 55, 66, 77, 88, 99)
print(tuple_data)
# elements from 3rd to 5th
# prints (33, 44, 55)
print(tuple_data[2:5])
# elements from start to 4th
# prints (11, 22, 33, 44)
print(tuple_data[:4])
# elements from 5th to end
# prints (55, 66, 77, 88, 99)
print(tuple_data[4:])
# elements from 5th to second last
# prints (55, 66, 77, 88)
print(tuple_data[4:-1])
# displaying entire tuple
print(tuple_data[:])
(11, 22, 33, 44, 55, 66, 77, 88, 99)
(33, 44, 55)
(11, 22, 33, 44)
(55, 66, 77, 88, 99)
(55, 66, 77, 88)
(11, 22, 33, 44, 55, 66, 77, 88, 99)
Finding Length of a Tuple
The length of a tuple can be found using the len()
function.
#Code for printing the length of a tuple
tuple_data =('Flutter', 'ReactNative')
print(len(tuple_data))
2
Membership tests in tuple
We can test if an item exists in a tuple or not using following operators
in: Checks whether an element exists in the specified tuple.
not in: Checks whether an element does not exist in the specified tuple.
# Membership test in tuple
my_tuple = ('g', 'l', 'a', 's', 's',)
# In operation
print('a' in my_tuple)
print('l' in my_tuple)
# Not in operation
print('p' not in my_tuple)
True
False
True
Iterating a tuple
You can also loop through the tuple values using a for
loop.
tuple_data = ("alpha","bravo","charlie")
for item in tuple_data:
print(item)
alpha
bravo
charlie
Converting list to a Tuple
# Code for converting a list and a string into a tuple
list1 =[0, 1, 2]
print(tuple(list1))
print(tuple('headphone')) # string 'headphone'
(0, 1, 2)
('h', 'e', 'a', 'd', 'p', 'h', 'o', 'n', 'e')
Above were some of the commands and explanation of what are tupple in python.