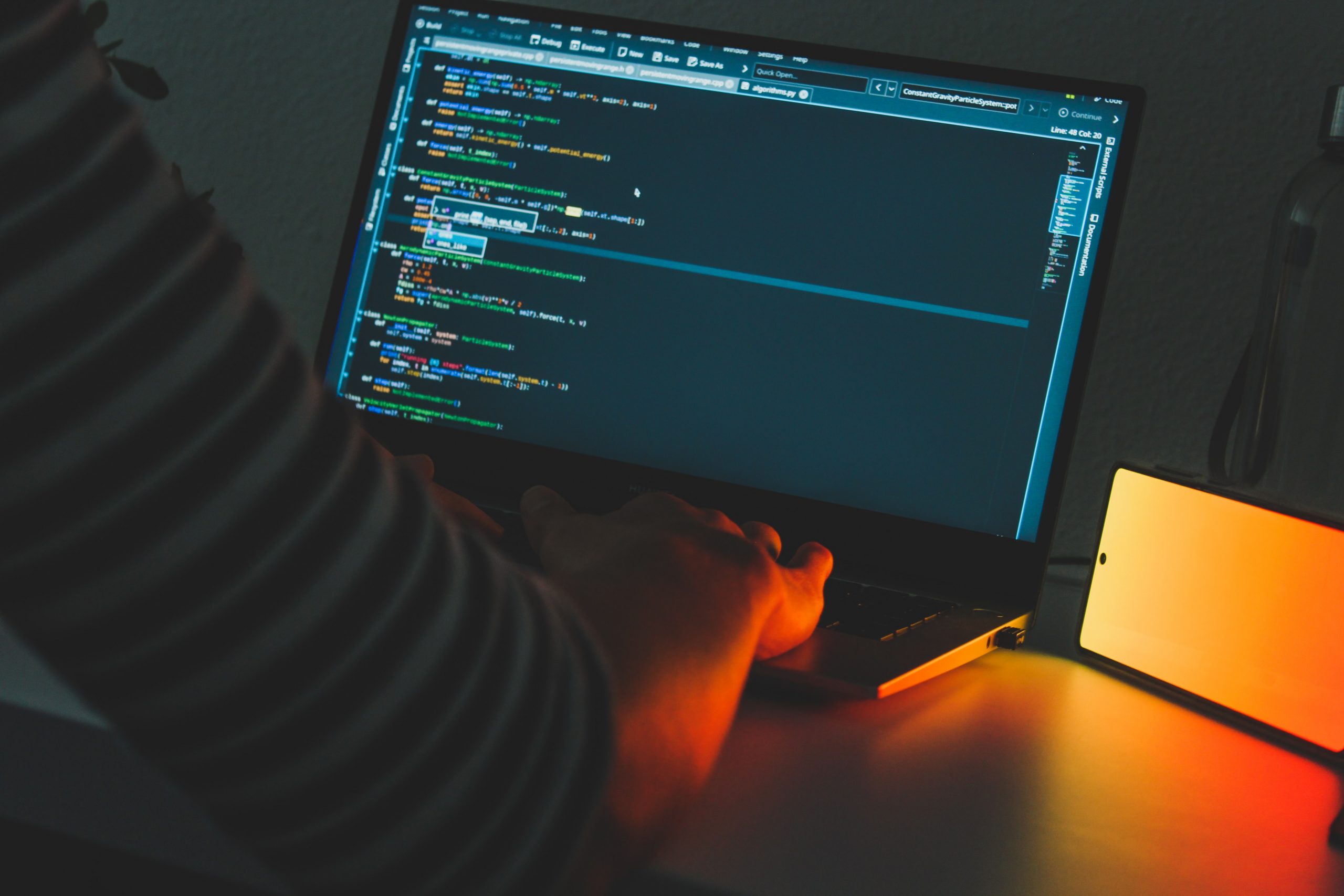
User inputs are vastly integrated into many programs as they serve as a valuable asset for the company or business. Developers use this user input method to interact with platform users so to either get the data directly from users or to evaluate specific results.
While most programs offer a dialog box to get some sort of input from users, in Python, you are provided with two built-in functions to get the input from the user’s keyboard i.e., input (Prompt) & raw_input (Prompt)
Input (): Using this user input function, Python automatically identifies whether the user has entered a number that is an integer, float & decimal, or a list & even a string. To put it in simple words, The Input () function first takes the user input and then evaluates the expression. If the provided input is wrong, a syntax error or an exception is raised in python.
# Python program showing a use of input()
val = input("Enter your value: ")
print(val)
Enter your value: 12345
12345
Here’s how input() function works
When this user input function is executed, the program flow halts until some kind of input is given by the user.
The output screen displays a message that may ask the user to input a value.
It’s important to note that, regardless of the data type printed by the user when to ask to input the value to continue the flow of the program, the output is always a string. That is even the user entered an integer, float, string, or list, the output will be always a string. To change the data type you need to use “Typecasting” in Python.
Here’s how you can check and change the input type using typecasting in python.
# Program to check input type in Python
num = input ("Enter number :")
print(num)
name1 = input("Enter name : ")
print(name1)
# Printing type of input value
print ("type of number", type(num))
print ("type of name", type(name1))
Enter number: 1
1
Enter name: techstricks
type of number <class 'str'>
type of name <class 'str'>
raw_input (): This function takes the input from the keyboard when asked to type the value and then converts it into a string. Doing this will return that exact variable in which we want to store the value.
The raw_input () function only works in the older versions of python such as; python 2. x and below.
# Python program showing a use of raw_input()
t = raw_input("Enter your name: ")
print t
Enter: techstricks
techstricks
Here we can see, t is a variable typed by the user while the execution of the program that will get our value as an output.
We can also use the raw_input() function to insert numeric values by using the typecasting method.